Basic usage¶
Basic process of face recognition¶
1. Monocular recognition¶
Monocular recognition is to use ordinary color camera to do liveness check, and then extract and compare the face features.
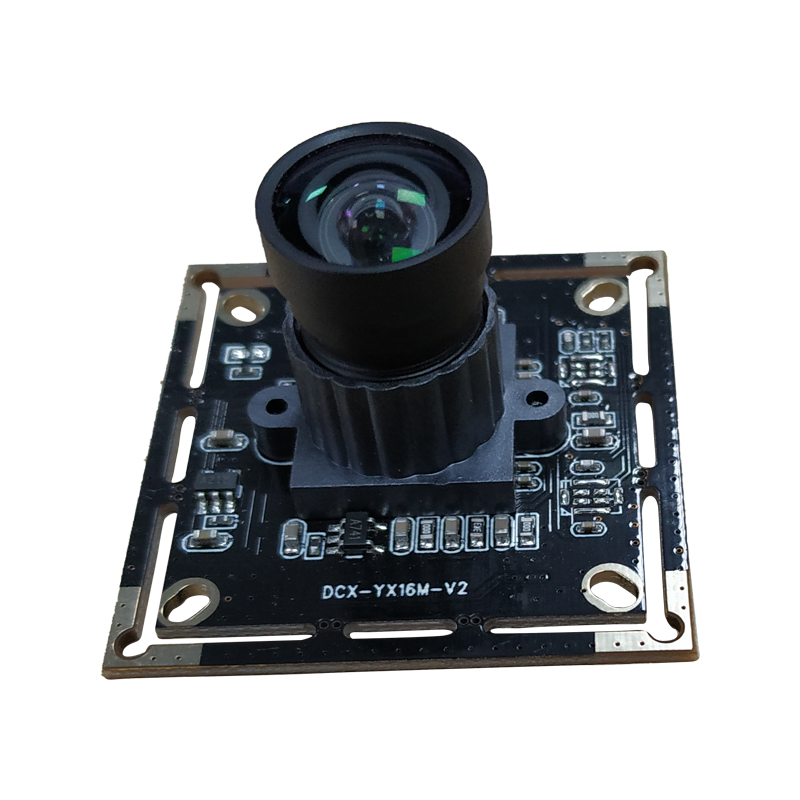
flow chart:
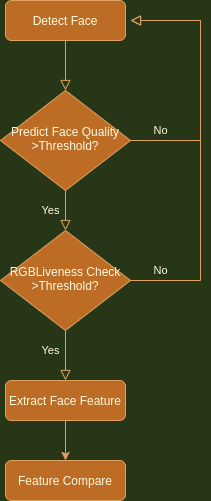
Pseudo code:
//Face detection and return box
Bbox faceBbox = dfaceDetect.detectionMax(frame);
//predict face quality
float qualityScor = dfaceAssessment.predictQuality(frame, faceBbox);
if(qualityScore < 0.45){
print("Low Quality");
return;
}
//RGB liveness check
float liveScore = dfaceRGBLiveness.liveness_check(frame, faceBbox);
if(liveScore < 0.45){
print("Fake Face");
return;
}
//extract face feature
byte[] feature = dfaceRecognize.extractFaceFeatureByImg(frame, faceBbox);
//Search for users with the highest similarity according to features
User matchedUser = findTopSimilarityUser(feature);
2. Binocular recognition¶
Binocular recognition is to use binocular camera (color + infrared) do liveness check, then extract face features and compare them.
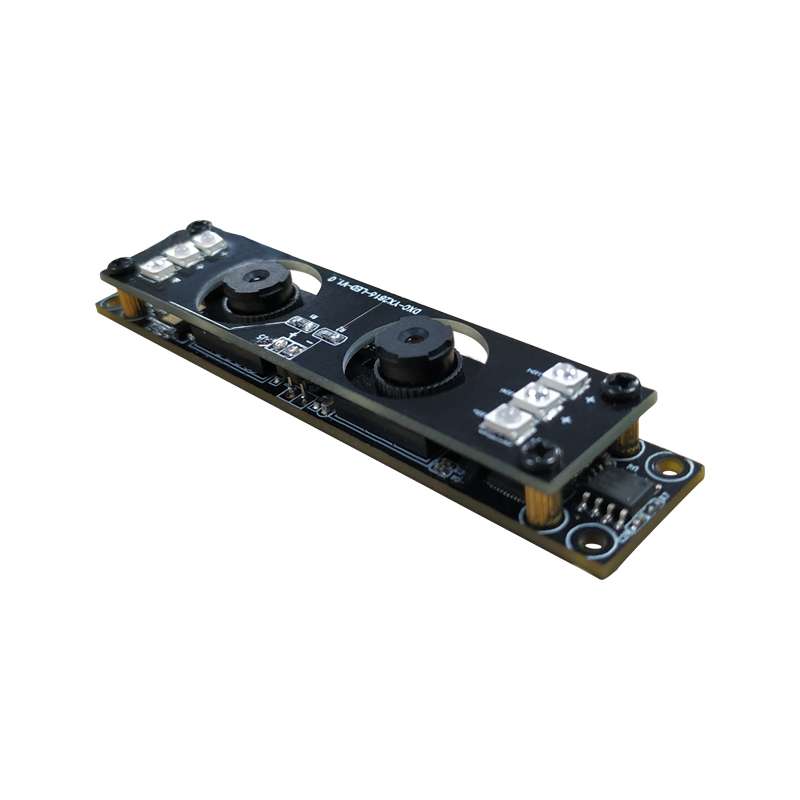
flow chart:
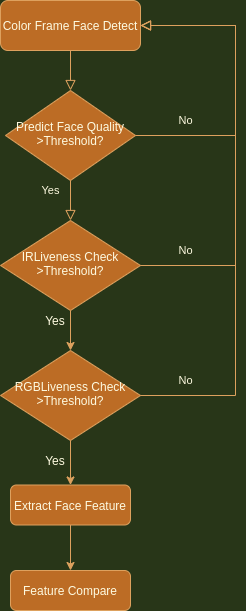
Pseudo code:
//The color camera detects the face and returns the face box
Bbox faceBboxColor = dfaceDetect.detectionMax(colorFrame);
//predict face quality
float qualityScor = dfaceAssessment.predictQuality(colorFrame, faceBboxColor);
if(qualityScore < 0.45){
print("Low Quality");
return;
}
//The ir camera detects the face and returns the face box
Bbox faceBboxIR = dfaceDetect.detectionMax(irFrame);
//NIR liveness check
float irLiveScore = dfaceNIRLiveness.liveness_check(faceBboxColor, faceBboxIR);
if(irLiveScore < 0.45){
print("Fake Face");
return;
}
//RGB liveness check
float colorLiveScore = dfaceRGBLiveness.liveness_check(colorFrame, faceBboxColor);
if(colorLiveScore < 0.45){
print("Fake Face");
return;
}
//extract face feature
byte[] feature = dfaceRecognize.extractFaceFeatureByImg(colorFrame, faceBboxColor);
//Search for users with the highest similarity according to features
User matchedUser = findTopSimilarityUser(feature);
Usage of DFACE modules¶
The SDK provides face detection, face recognition, face pose, liveness detect and other independent functions to provide corresponding modules, users can flexibly call according to their own needs.
1. DfaceDetect¶
DfaceDetect is a face detection module, which can realize multi-person detection, maximum face detection.
//set min size dfaceDetect.SetMinSize(60); //Detect face and return to face box list List<Bbox> faceBboxs = dfaceDetect.detection(frame, false);
2. DfaceTrack¶
DfaceTrack is a face tracking module that can implement real-time multi-face tracking.
//start track dfaceTrack.start(); while(frame){ //detect face List<Bbox> faceBboxs = dfaceDetect.detection(frame, false); //Tracking faces requires the above frame input for detecting faces List<Bbox> trackBoxs = dfaceTrack.update(frame, faceBboxs); } //stop track dfaceTrack.stop();
Warning
Tracking is an estimation judgment, so the returned position information may exceed the camera screen range. It is best to use it alone to make a judgment with the camera screen range to avoid cross-border access.
3. DfaceRecognize¶
DfaceRecognize is a face recognition module, mainly used for face feature extraction. .. code:: java
//set min size List<Bbox> bbox = dfaceDetect.detectionMax(frame, true); //Extract face features byte[] feature1 = dfaceRecognize.extractFaceFeatureByImg(frame, bbox);
4. DfaceCompare¶
DfaceCompare is often used for face feature comparison.
//Extract face features
byte[] feature1 = dfaceRecognize.extractFaceFeatureByImg(frame, bbox1);
byte[] feature2 = dfaceRecognize.extractFaceFeatureByImg(frame, bbox2);
//n feature
byte[][] feature_n;
//n feature id
long[] idx_n;
//Feature comparison (1:1)
float similarity = dfaceCompare.similarityByFeature(feature1, feature2);
5. DfacePose¶
DfacePose is a face pose module, which is mainly used to detect landmarks and poses of face 98.
//set min size List<Bbox> bbox = dfaceDetect.detectionMax(frame, true); //detect landmarks List<FaceLandmark> poses = dfacePose.predictPose(frame, Arrays.asList(bbox));Note
Euler angles Introduction Euler angles
yaw: Face view, turning the face to the right is a positive angle, turning the face to the left is a negative angle (unit degree)
pitch: Face view, downward is a positive angle, upward is a negative angle (unit degree)
roll: Face view, roll right is positive, and roll left is negative (unit degree)
t_x: Camera view, the deviation to the right of the camera is positive, and the left is negative (unit meters)
t_y: Camera view, the deviation from the camera is positive, and the negative is negative (unit meters)
t_z: Camera view. The farther away from the camera, the larger the value. This value is closely related to the focal length of the camera. The actual generation environment needs to set the focal length parameters
6. DfaceRGBLiveness¶
DfaceRGBLiveness is an RGB liveness detection module, commonly used for liveness detect.
//Liveness detect needs to switch back to the original image, minimize the information loss caused by resize float score = dfaceRGBLiveness.liveness_check(frame, box);
7. DfaceNIRLiveness¶
DfaceNIRLiveness is a near-infrared liveness detection module, commonly used for infrared liveness detect.
//Liveness detect
float score = dfaceNIRLiveness.liveness_check(ir_box, color_box);
8. DfaceAssessment¶
DfaceAssessment is a face quality assessment module.
//质量判断
float score = dfaceAssessment.predictQuality(frame, box);
9. DfaceTool¶
DfaceTool tool class contains some image processing, feature conversion and database processing methods.